How to Build mobile Applications Using React Native
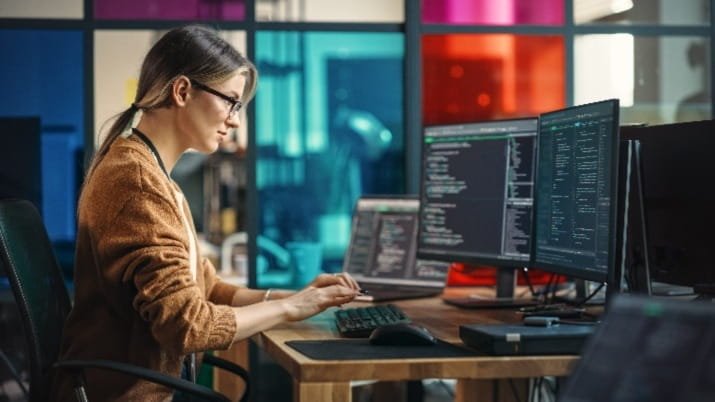
React Native has become a popular framework for building cross-platform apps due to its ability to write code once and deploy it on both iOS and Android. This makes it highly efficient for top React Native app developers and businesses aiming to reach a broad audience. In this guide, we will walk you through the steps and tools needed to build an app using React Native.
What is React Native App Development?
React Native app development refers to using the React Native framework to build mobile applications that can run on multiple platforms, primarily iOS and Android, from a single codebase. Developed by Facebook, React Native allows developers to use JavaScript and React (a popular web development library) to create native mobile applications.
The framework bridges the gap between web and mobile development by rendering real native components, rather than web views, which results in smoother performance and user experience. React Native enables access to native APIs through JavaScript, allowing them to integrate platform-specific functionalities and components within their apps. Its cross-platform capability reduces development time and costs, making it a popular choice for businesses looking to deploy apps on multiple platforms efficiently.
Building React Native Apps: The Process
Here is the complete guide to build your first react native app:
1. Setting Up Your Environment
The first step in building an app using React Native is setting up your development environment. This process varies slightly depending on whether you are developing for iOS or Android, but the general tools you’ll need include:
- Node.js: React Native relies on Node.js, so install the latest version. You can download it from Node.js.
- Watchman: A tool developed by Facebook for watching filesystem changes. This is optional but recommended for better performance.
- React Native CLI: Install the React Native CLI globally by running the command:
npm install -g react-native-cli - Android Studio (for Android development): This includes the Android SDK, virtual device, and build tools.
- Xcode (for iOS development): If you’re developing for iOS, you’ll need a Mac with Xcode installed, as it includes all necessary iOS tools and simulators.
After the setup, create a new React Native project by running:
npx react-native init ProjectName
2. Understanding React Native Components
React Native allows you to build mobile apps using JavaScript and React. The core building blocks are React components, which are reusable pieces of code responsible for rendering UI. Components like View, Text, and Button are examples of the basic building blocks in React Native.
Using these components, you can design the layout of your app just like you would with HTML and CSS, but React Native also offers native UI rendering. This means that although you write the app in JavaScript, it will render native components like those seen in Swift for iOS or Java/Kotlin for Android.
3. Building the User Interface (UI)
React Native uses a flexbox layout system, which is a highly flexible way to structure your app’s UI. You can stack components vertically or horizontally, making it easy to create responsive layouts.
A simple layout might look like this:
javascript
Copy code
import React from ‘react’;
import { View, Text, Button } from ‘react-native’;
const App = () => {
return (
<View style={{ flex: 1, justifyContent: ‘center’, alignItems: ‘center’ }}>
<Text>Hello, React Native!</Text>
<Button title=”Click me” onPress={() => alert(‘Button clicked!’)} />
</View>
);
};
export default App;
4. Using Third-Party Libraries
One of the significant advantages of React Native is its vast ecosystem of third-party libraries. From navigation libraries like React Navigation to UI component libraries like NativeBase and React Native Elements, you can leverage these resources to speed up development.
To install a library, you can use npm or yarn. For example, to install React Navigation:
bash
Copy code
npm install @react-navigation/native
Then you can start adding multiple screens and implement navigation logic between them.
5. State Management
For managing state in your app, React Native supports tools like Redux and React Context API. Redux is highly popular in large-scale apps due to its predictable state management pattern, while React Context works well for smaller apps.
With Redux, you can centralize your app’s state and make data available globally across all components.
6. Testing and Debugging
React Native provides tools like Metro, a JavaScript bundler that ensures your app runs efficiently. To debug your app, you can use tools like the React Native Debugger, which lets you inspect elements, view console logs, and debug your app’s state.
You can test your app either on a physical device or using Android and iOS emulators. For iOS, run:
bash
Copy code
npx react-native run-ios
For Android, run:
bash
Copy code
npx react-native run-android
7. Deploying the App
Once your app is complete and tested, you’ll need to deploy it to either the App Store (for iOS) or Google Play (for Android). For iOS deployment, you will need a Mac to access Xcode and use tools like TestFlight to beta test the app. Android deployment can be handled using Android Studio or Google Play Console.
Conclusion
Building apps with React Native offers numerous benefits, from writing a single codebase for multiple platforms to leveraging a vast ecosystem of libraries. For top React Native app developers, the framework provides flexibility and scalability, making it ideal for both small-scale apps and large, complex projects. As React Native continues to evolve, it remains a popular choice for businesses aiming to streamline mobile app development across iOS and Android platforms.
FAQs
- Is React Native enough to build an app?
Yes, React Native is sufficient to build fully functioning mobile apps that work across platforms. It offers access to both native and JavaScript APIs for mobile-specific functionality. - Can I build a web app with React Native?
React Native is primarily for mobile apps, but you can use React Native for Web to adapt your mobile app code for web deployment. - Is React Native frontend or backend?
React Native is a frontend framework used to create the user interface of mobile apps. For backend services, developers typically use Node.js, Firebase, or other server-side technologies. - Is React Native easy to learn?
For developers familiar with JavaScript and React, React Native is relatively easy to pick up. It shares many concepts with React but also requires knowledge of mobile-specific APIs and environments. - Is React Native full stack?
React Native is not considered full stack. It handles only the frontend part of app development, while backend services and databases are usually built using other technologies.