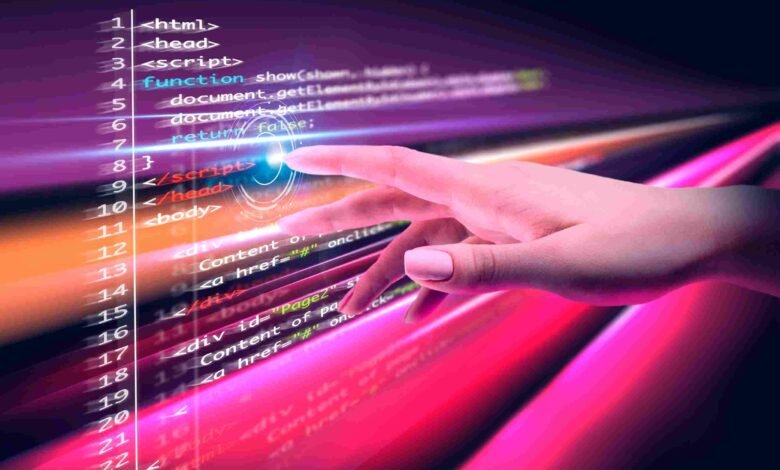
Handling Exceptions in Pytest: Strategies for Error Testing
When it comes to software testing Automation with Python , using Selenium WebDriver for browser automation is a popular choice. However, ensuring your tests can handle exceptions gracefully is crucial for maintaining stable and reliable test suites. In this article, we’ll explore various strategies for handling exceptions in Pytest, specifically focusing on error testing in Selenium WebDriver and Python automation testing.
Table of Contents
Sr# | Headings |
1 | Introduction to Exception Handling in Pytest |
2 | Common Exceptions in Selenium WebDriver |
3 | Using Try-Except Blocks for Error Handling |
4 | Handling Specific Exceptions with Except Blocks |
5 | Using Assert Statements for Error Verification |
6 | Implementing Custom Exception Handlers |
7 | Best Practices for Exception Handling in Pytest |
8 | Conclusion |
9 | FAQs |
Introduction to Exception Handling in Pytest
In any programming language, including Python, exceptions are unexpected events that disrupt the normal flow of code execution. When writing automated tests, it’s essential to anticipate and handle these exceptions to prevent test failures. Pytest, a popular testing framework in python for automation testing , provides robust support for handling exceptions, making it an excellent choice for testing Selenium WebDriver-based applications.
Common Exceptions in Selenium WebDriver
When working with selenium webdriver python , several common exceptions can occur, such as NoSuchElementException, ElementNotVisibleException, TimeoutException, and StaleElementReferenceException. Each of these exceptions signifies a different issue that may arise during test execution, such as an element not being found on the page, an element being hidden or obscured, a timeout while waiting for an element, or a reference to an element that is no longer valid.
Using Try-Except Blocks for Error Handling
One of the most fundamental strategies for handling exceptions in Python is using try-except blocks. By wrapping code that might raise an exception in a try block and catching the exception in an except block, you can gracefully handle the error without causing your test to fail. Here’s an example of using a try-except block to handle a NoSuchElementException in Selenium WebDriver:
python
Copy code
from selenium.common.exceptions import NoSuchElementException
try:
element = driver.find_element_by_id(“my_element”)
except NoSuchElementException:
print(“Element not found!”)
Handling Specific Exceptions with Except Blocks
In addition to catching generic exceptions, such as Exception, you can also catch specific exceptions to handle different types of errors differently. This allows you to tailor your error handling logic to the specific circumstances of each exception. For example, you might want to handle a TimeoutException by retrying the operation, while handling a NoSuchElementException by logging an error and continuing with the next test step.
Using Assert Statements for Error Verification
Another approach to handling exceptions in Pytest is to use assert statements to verify that certain conditions are met. For example, you can use assert statements to check if an element is present on the page before interacting with it:
python
Copy code
assert driver.find_element_by_id(“my_element”).is_displayed(), “Element is not visible!”
If the condition specified in the assert statement is not met, the test will fail, and the error message provided will be displayed in the test report.
Implementing Custom Exception Handlers
In some cases, the built-in exception handling mechanisms in Python and Pytest may not be sufficient for your needs. In such cases, you can implement custom exception handlers to handle specific types of errors in a more tailored manner. For example, you might create a custom exception handler to handle authentication errors in your application:
python
Copy code
class AuthenticationError(Exception):
pass
try:
authenticate_user()
except AuthenticationError:
print(“Authentication failed!”)
Best Practices for Exception Handling in Pytest
When handling exceptions in Pytest, it’s essential to follow best practices to ensure your tests are robust and reliable. Some best practices include:
- Use specific exception handling: Catch specific exceptions rather than generic ones to handle errors more precisely.
- Keep exception handling code separate: Separate your exception handling code from your test logic to keep your tests clean and maintainable.
- Use assert statements: Use assert statements to verify expected conditions and fail the test if they are not met.
- Implement retries: Implement retry mechanisms for operations that may fail intermittently due to timing issues.
Conclusion
In conclusion, handling exceptions in Pytest is crucial for ensuring the stability and reliability of your automated tests. By following the strategies outlined in this article, you can effectively handle exceptions in Automation Testing with Python and Python automation testing, making your tests more robust and reliable.
FAQs
Q: How do I handle a TimeoutException in Selenium WebDriver?
A: You can handle a TimeoutException by using a try-except block to catch the exception and implement a retry mechanism or log an error message.
Q: Can I use assert statements to handle exceptions in Pytest?
A: Yes, you can use assert statements to verify that certain conditions are met and fail the test if they are not.
Q: What is the advantage of using specific exception handling in Pytest?
A: Using specific exception handling allows you to handle different types of errors differently, making your error handling logic more precise and tailored to the specific circumstances of each exception.
Q: How can I implement custom exception handlers in Python?
A: You can implement custom exception handlers by defining a new exception class that inherits from the base Exception class and then using a try-except block to catch instances of your custom exception.
Q: What are some best practices for exception handling in Pytest?
A: Some best practices for exception handling in Pytest include using specific exception handling, keeping exception handling code separate from test logic, using assert statements, and implementing retries for operations that may fail intermittently.
Q: How does Pytest help in writing readable code ?
A: Pytest can help enforce coding standards and best practices in selenium webdriver , ensuring your code is consistent and easy to follow.
Q: Can Pytest be used to check code coverage?
A: Yes, Pytest can generate code coverage reports using plugins like pytest-cov in Automation with Python.
Q: What are some popular Pytest plugins for code analysis?
A: Some popular Pytest plugins for code analysis include pytest-flake8, pytest-mypy, and pytest-black.
Q: How can I enforce coding standards using Pytest?
A: You can use Pytest to write test cases that check for adherence to coding standards, such as variable naming conventions and documentation.
Q: What are some best practices for integrating code quality checks into my development process?
A: Some best practices include starting early, using meaningful test names, keeping tests small and focused, and regularly reviewing and refactoring your code in selenium webdriver python.